Python
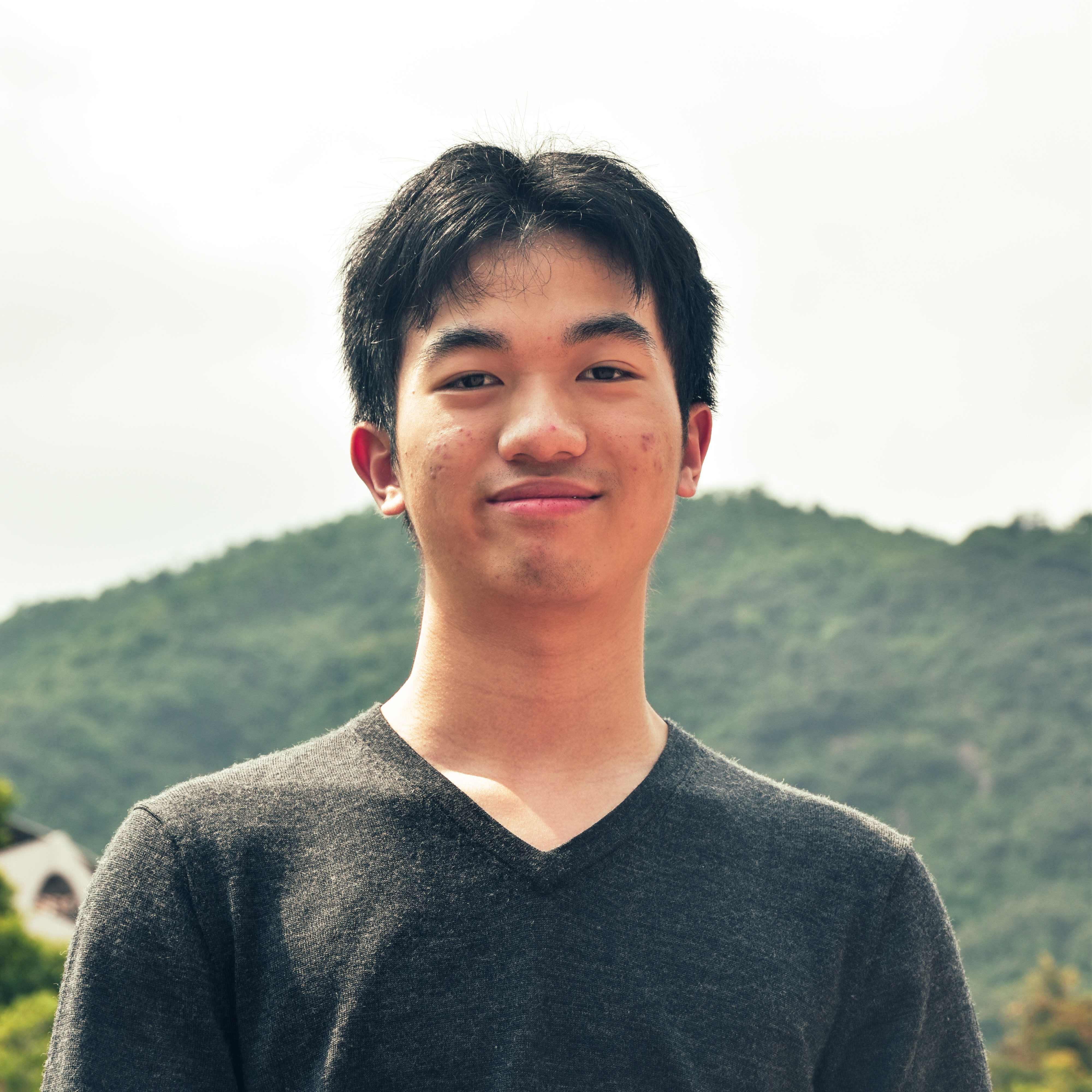
Peter Chow | July 30 - August 28, 2020 | Python | Selenium | OpenCV | Tkinter
A video of the Instagram like bot running.
The Idea
As university gets closer and closer, I decided to check out Python as it is the main programming language for my freshman year. Python is a great programming language especially for automating things. There were several things that I really wanted to automate on my computer, thus this side project was born. The things I made during my python adventure are an Instagram bot to like Instagram posts for my friend Kasen Yip, OpenCV face detection, a workflow for automating moving files in the Downloads folder, and a simple Tkinter program for saving passwords.
Instagram Bot
The idea of creating this bot was coined when I went camping with my friends. My friend Kasen Yip wanted to create a bot that would like new posts on #fitness on Instagram to attract more followers and spread awareness with his fitness Instagram account. So I made a bot for him.
Selenium is a great module that allows for automated web browsing that can do anything you want. Essentially, you would have to script the "bots" every single action such as clicking a button, typing into an input element, going to another page, and more. The "bot" of selenium is controlled by chromedriver, a tool for automating web browsing. This is easy as we can use methods from the selenium module such as click(), send_keys(), and get(). Also, to find certain elements, we can find it in multiple ways. The method is normally called "find_element_by_xxx", where xxx could be different HTML attributes, CSS selector, or a full XPath. The google dev tool comes in handy when trying to pinpoint what element the web driver should use.
Since Instagram strongly discourages against bots, I had to use the time module to mimic human behavior. As a bot would do things faster than a human can, the time.sleep() method should be called. This method stops all execution for the given amount of seconds. This mimics a human pause.
As I want this program to be run every hour, Crontab is a great solution. Instead of having to manually run the python script, I can schedule the action of running a script every hour. I also stumbled upon Vim, a command-line text editor for macOS. Vim is used to edit the Crontab file and is fairly useful.
The Github gist below shows a snippet of the code.
OpenCV Face Detection
A video of the OpenCV face detection program running.
I also messed around with the OpenCV module. Although I don't really know how it all works, it is still useful and interesting nonetheless.
Automating moving files in the downloads folder
This was fairly easy to do if you use the os and shutil module. Since I only learned the beginner concepts in Python, I had to relearn many things in Python.
Essentially, I utilized the mac's Automator that would listen to changes to a folder and run a shell script. The shell script will then run the python script which will do the sorting in my downloads folder. Surprisingly, this method works really well.
The sorting method that is used to differentiate between the types of files is put in a var.py file. By reading the file extension of every file inside of the folder, the move() method in the shutil module can easily move the file to another directory given two parameters. Since the source code is fairly short, the whole code is in the Github gist below.
Tkinter program for saving passwords
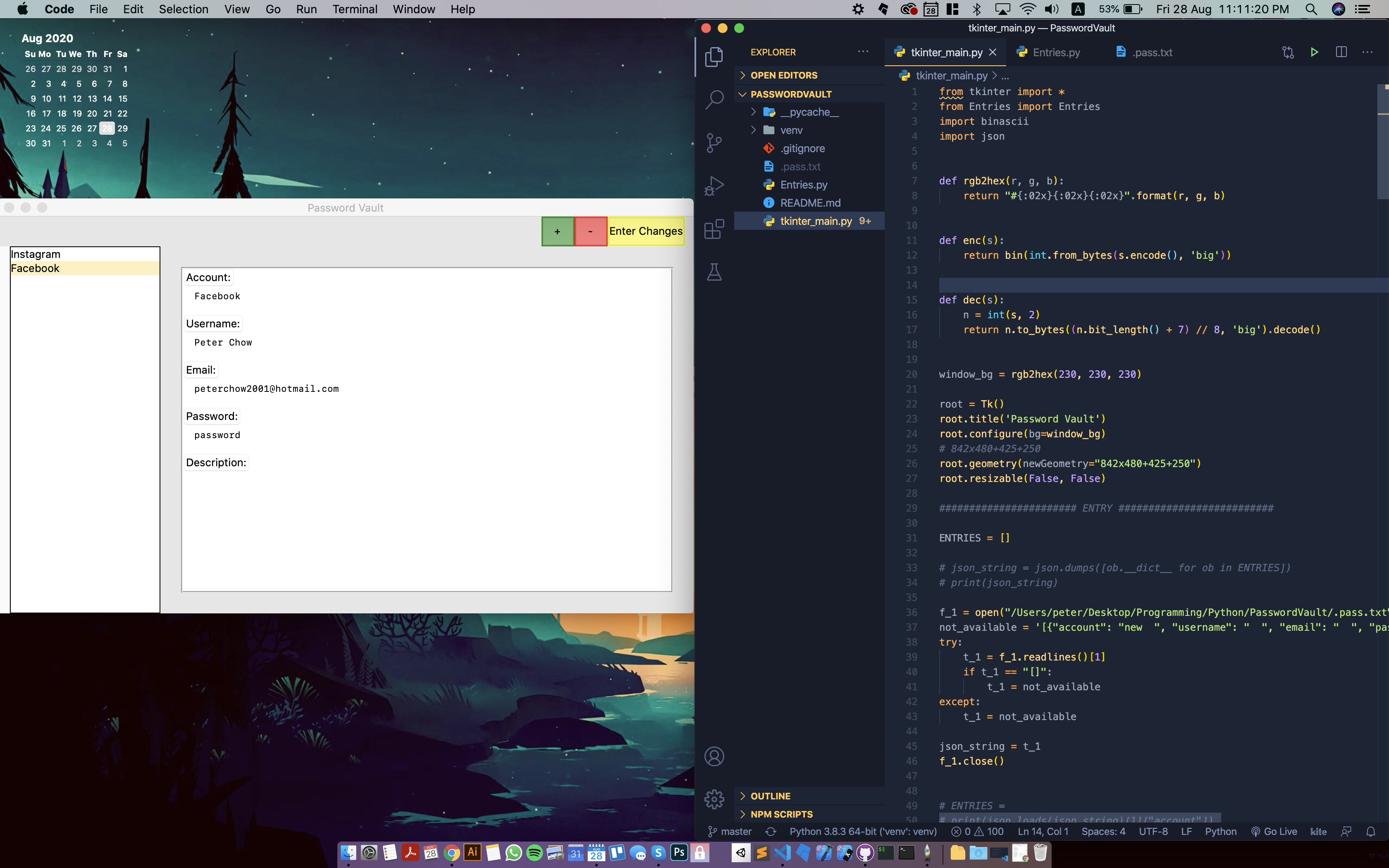
My setup. (Program left, vscode right)
My obsession with password managers got the better of me and I decided to make another program to put passwords in. Tkinter is a module for creating a GUI, or a graphical user interface. This will often have some back-end code following it. Although it doesn't look great as it wasn't made to look good, it is still an important module to learn for python. I plan to use ElectronJS in the future to create a desktop application that looks a lot nicer.
Tkinter shares many similarities with Flutter. Widgets class="projects-bold-text">Widgets like Text(), button(), and more are what make up the UI. There is also the basic parent/child concept here similar to Flutter and HTML.
I used each password entry as an object with the necessary parameters. This is important as I can display the parameters of the class easily. For storing the entries, I converted the entry objects into JSON, then into binary. This makes it harder for people to see the passwords. Also, the file with the stored entries is a hidden file that starts with a period.
Similar to automating moving files, I also used Automator for this. I created an application with automator that would run a shell script that would then run the python script.